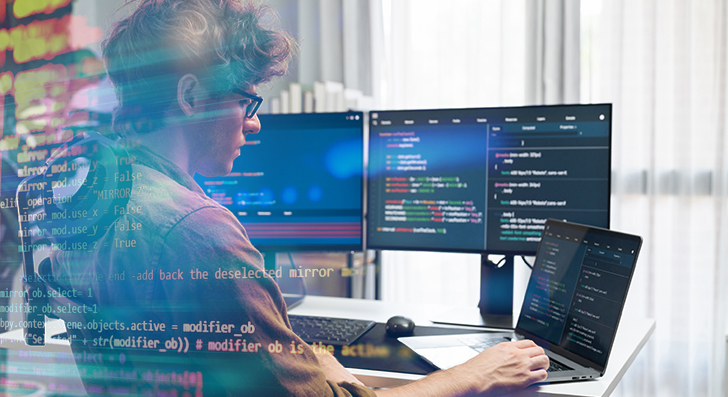
Scalability usually means your application can take care of development—more buyers, additional knowledge, and even more visitors—without breaking. For a developer, creating with scalability in your mind saves time and tension afterwards. Right here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element of your prepare from the start. A lot of purposes fall short every time they expand speedy due to the fact the first design and style can’t tackle the extra load. For a developer, you have to Consider early regarding how your program will behave stressed.
Start by planning your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These styles break your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle a million end users or merely 100? Pick the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial point is to avoid hardcoding assumptions. Don’t create code that only operates beneath recent problems. Contemplate what would transpire If the person base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that assist scaling, like concept queues or occasion-pushed devices. These assist your app handle more requests without getting overloaded.
When you Establish with scalability in your mind, you are not just getting ready for achievement—you are lowering long term headaches. A perfectly-prepared technique is easier to maintain, adapt, and mature. It’s superior to arrange early than to rebuild later on.
Use the correct Database
Deciding on the appropriate database is a critical Section of developing scalable programs. Not all databases are built a similar, and utilizing the Incorrect you can sluggish you down or even induce failures as your app grows.
Start by knowledge your facts. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. They're potent with associations, transactions, and consistency. In addition they help scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your facts is more adaptable—like consumer exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally much more quickly.
Also, think about your read through and write patterns. Will you be doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a heavy compose load? Check into databases that can manage significant generate throughput, or perhaps function-dependent details storage systems like Apache Kafka (for momentary details streams).
It’s also smart to Feel forward. You might not will need Highly developed scaling features now, but choosing a database that supports them indicates you gained’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on database general performance when you mature.
To put it briefly, the ideal databases relies on your application’s framework, pace wants, And the way you count on it to improve. Acquire time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make productive logic from the start.
Start by producing clean up, uncomplicated code. Keep away from repeating logic and remove just about anything unwanted. Don’t choose the most complex Option if an easy one will work. Maintain your capabilities shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes way too lengthy to operate or makes use of too much memory.
Future, have a look at your databases queries. These typically gradual factors down more than the code by itself. Make sure Every single query only asks for the information you truly require. Steer clear of Pick out *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Specially throughout big tables.
When you recognize a similar information currently being asked for again and again, use caching. Retailer the effects temporarily making use of instruments like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app additional economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with 100 information may well crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional people plus more targeted visitors. If all the things goes through one server, it will swiftly become a bottleneck. That’s exactly where load balancing and caching come in. These two applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors throughout various servers. In place of just one server accomplishing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this very easy to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical information all over again—like a product page or maybe website a profile—you don’t must fetch it from the databases each time. You are able to provide it from your cache.
There's two widespread types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents near the user.
Caching cuts down databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does transform.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your application take care of more consumers, continue to be quick, and Get well from problems. If you plan to improve, you'll need both.
Use Cloud and Container Equipment
To build scalable applications, you may need applications that let your app increase conveniently. That’s in which cloud platforms and containers can be found in. They offer you flexibility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to rent servers and solutions as you may need them. You don’t should buy hardware or guess potential ability. When targeted visitors improves, you can include far more methods with just a couple clicks or mechanically making use of car-scaling. When targeted traffic drops, you may scale down to economize.
These platforms also give services like managed databases, storage, load balancing, and security resources. You can concentrate on creating your app instead of running infrastructure.
Containers are A further key Software. A container offers your app and everything it really should operate—code, libraries, options—into one particular unit. This makes it easy to maneuver your app among environments, from your notebook to your cloud, with no surprises. Docker is the most popular Instrument for this.
Once your application makes use of a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If 1 section of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into products and services. It is possible to update or scale components independently, and that is great for general performance and trustworthiness.
In a nutshell, using cloud and container instruments indicates you could scale rapidly, deploy easily, and Get well rapidly when challenges occur. If you prefer your app to improve with out boundaries, begin working with these tools early. They preserve time, reduce hazard, and enable you to continue to be focused on creating, not correcting.
Monitor Almost everything
For those who don’t check your software, you received’t know when items go Erroneous. Checking assists the thing is how your application is carrying out, place difficulties early, and make better choices as your application grows. It’s a crucial Component of setting up scalable methods.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just keep an eye on your servers—observe your application much too. Keep an eye on how long it will take for customers to load internet pages, how often errors occur, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential difficulties. As an example, Should your response time goes over a limit or a company goes down, you'll want to get notified straight away. This allows you deal with difficulties rapidly, normally right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh feature and find out a spike in problems or slowdowns, you are able to roll it again in advance of it triggers genuine destruction.
As your application grows, visitors and data raise. Without having checking, you’ll miss out on signs of difficulty right until it’s way too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for major organizations. Even compact apps will need a strong Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Create applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and build wise.